Street Smart
For the final project in “Internet of Things” last spring, our team created an API, web app, and Raspberry Pi car sensor. The Pi utilizes an on-board Diagnostics (OBD) interface and the Overpass API to enable in-cabin alerts when speeding is detected. All events are forwarded to Firebase, and an Express API and React App hosted on Heroku provide reports and visualizations of driving habits and vehicle health.
Showcase Poster • GitHub
(We use the free tier of Heroku, meaning the app sleeps every 30 minutes. You might have to wait a moment for the app to spin up upon initial load: Street-Smart.xyz 👍)
The Front End
Harsh Patel and I worked on the Express API and React interface in order to demonstrate viewing sensor data on a “real-time” map. I tore apart React Shards, a well styled site template from Design Revision, to throw together an interface quickly. I was most proud of my implementation of the real-time map, as that component card was created from scratch using our API, and React Leaflet.
Car Travel Simulation
To have realistic test data, and to demo to our class, I created a script for generating car travel data. It simulates driving locations and speeds along a given route of coordinates based on adjustable driver tendencies, and uploads to Firebase in time accurate spacing, just like our in-car sensor. It was great to implement async javascript, but going forward I really want to use proper modules now that I understand how they work in ES6 a bit better. Too many functions in one file. This script was created overnight because we were having so many difficulties getting accurate location data for our sensor, so I’m proud of what I was able to do in that amount of time.
- javascript
1 | require('dotenv').config(); |
In-Car Sensor + Alerts
Our Raspberry Pi ran a script to collect GPS location and utilized the Overpass API to fetch the speed limit of that location, pair them, and publish them to our database. Additionally, if an instance of speeding was detected, the Raspberry Pi lit up an LED to alert the driver.
Awards
Our presentation and live demo earned us one of 3 spots for IoT in “Modern Marvels”, the university’s showcase for top projects completed throughout the year.
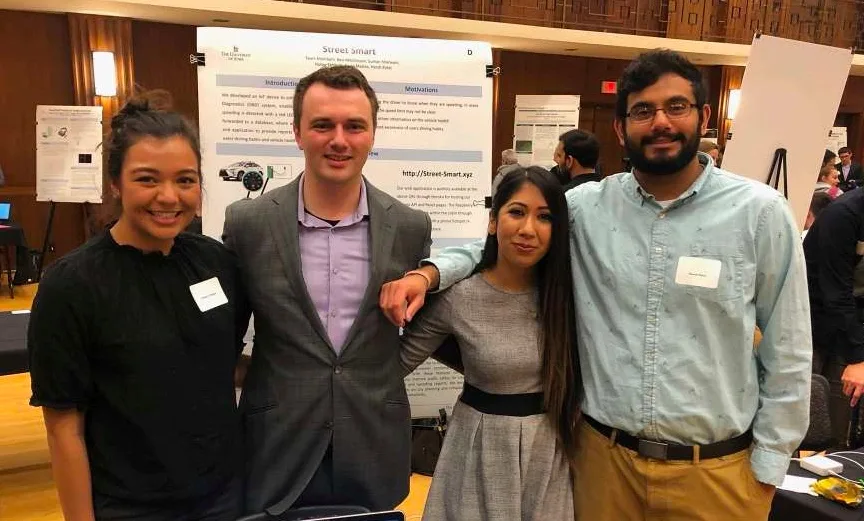
Improvements
- Next time I build an API, I’d like to try GraphQL. Our client fetched much more data then was necessary, and did client-side filtering that could have been accomplished in the API layer.
- We only mocked the user account and lacked any sort of authentication on both the database or front end. Integrating tools like OAuth is something I need to get a handle on soon.